Object tracking in videos – Exploring Video Data
Concept: Object tracking involves locating and following objects across consecutive video frames. It is essential for applications such as surveillance, human-computer interaction, and autonomous vehicles.
Tools: OpenCV (for tracking algorithms such as KLT and MedianFlow).
Here’s a brief overview of the steps in the object tracker code:
Tracker initialization: Create a Kernelized Correlation Filters (KCF) object tracker using OpenCV’s cv2.TrackerKCF_create():
import cv2
# Create a KCF tracker
tracker = cv2.TrackerKCF_create()
Video capture: Open a video file (sample_video.mp4) using cv2.VideoCapture:
# Read a video file
cap = cv2.VideoCapture(‘./PacktPublishing/DataLabeling/ch08/video_dataset/CricketBowling.mp4’)
Select object to track: Read the first frame and use cv2.selectROI to interactively select the object to be tracked:
# Read the first frame
ret, frame = cap.read()
bbox = cv2.selectROI(‘Select Object to Track’, frame, False)
We get the following result:
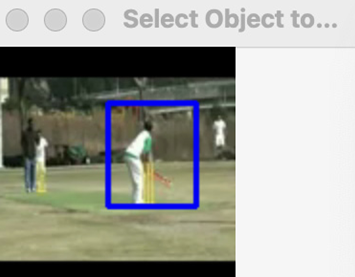
Figure 8.9 – Select object to track
Initialize tracker: Initialize the KCF tracker with the selected bounding box (bbox) in the first frame:
tracker.init(frame, bbox)
Object tracking loop: Iterate through subsequent frames in the video:
while True:
ret, frame = cap.read()
if not ret:
break
Update tracker: Update the tracker with the current frame to obtain the new bounding box of the tracked object:
# Update the tracker
success, bbox = tracker.update(frame)
Draw bounding box: If the tracking is successful, draw a green bounding box around the tracked object in the frame.
if success:
p1 = (int(bbox[0]), int(bbox[1]))
p2 = (int(bbox[0] + bbox[2]), int(bbox[1] + bbox[3]))
cv2.rectangle(frame, p1, p2, (0, 255, 0), 2)
Display tracking result: Show the frame with the bounding box in a window named ‘Object Tracking’ using cv2.imshow:
cv2.imshow(‘Object Tracking’, frame)
We see the following result:
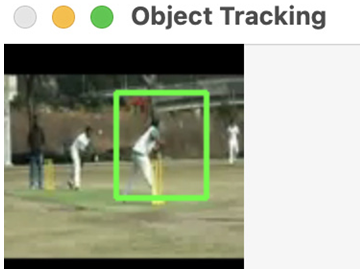
Figure 8.10 – Object tracking
Exit condition: Break the loop upon pressing the Esc key (cv2.waitKey):
# Break the loop on ‘Esc’ key
if cv2.waitKey(30) & 0xFF == 27:
break
Cleanup: Release the video capture object and close all OpenCV windows:
cap.release()
cv2.destroyAllWindows()
This code demonstrates a basic object-tracking scenario where a user selects an object in the first frame, and the KCF tracker is used to follow and draw a bounding box around that object in subsequent frames of the video.
Leave a Reply