A hands-on example to label video data segmentation using the Watershed algorithm – Labeling Video Data-2
The overall purpose of these steps is to preprocess the image and create a binary image (sure_bg) that serves as a basis for further steps in the watershed algorithm. It helps to distinguish the background from potential foreground objects, contributing to the accurate segmentation of the image:
Apply the distance transform to identify markers
dist_transform = cv2.distanceTransform(opening, cv2.DIST_L2, 5)
_, sure_fg = cv2.threshold(dist_transform, \
0.7*dist_transform.max(), 255, 0)
The distance transform is applied to the opening result. This transform calculates the distance of each pixel to the nearest zero (background) pixel. This is useful for identifying potential markers. The result is stored in dist_transform.
A threshold is applied to the distance transform, creating the sure foreground markers (sure_fg). Pixels with values higher than 70% of the maximum distance transform value are considered part of the foreground:
Combine the background and foreground markers
sure_fg = np.uint8(sure_fg)
unknown = cv2.subtract(sure_bg, sure_fg)
Apply the watershed algorithm to label the regions
_, markers = cv2.connectedComponents(sure_fg)
markers = markers + 1
markers[unknown == 255] = 0
markers = cv2.watershed(frame, markers)
In this code snippet, the markers = markers + 1 operation increments the values in the markers array by 1. In the watershed algorithm, markers are used to identify different regions or basins. By incrementing the marker values, you create unique labels for different regions, helping the algorithm distinguish between them.
markers[unknown == 255] = 0 sets the markers to 0, where the unknown array has a value of 255. In watershed segmentation, the unknown regions typically represent areas where the algorithm is uncertain or hasn’t made a decision about the segmentation. Setting these markers to 0 indicates that these regions are not assigned to any particular basin or region. This is often done to prevent the algorithm from over-segmenting or misinterpreting uncertain areas.
In summary, these operations are part of the process of preparing the marker image for the watershed algorithm. The incrementation helps to assign unique labels to different regions, while the second operation helps handle uncertain or unknown regions. The specifics may vary depending on the implementation, but this is a common pattern in watershed segmentation:
Colorize the regions for visualization
frame[markers == -1] = [0, 0, 255]
labeled_frames.append(frame)
Step 5: save the segmented frame to output directory.
and print the segmented frame.
Save the first segmented frame to the output folder
cv2.imwrite(‘<your_path>/datasets/Ch9/Kinetics/watershed/segmentation.jpg’, labeled_frames[0])
Now, let’s print the first segmented video frame:
plt.imshow(cv2.cvtColor(labeled_frames[0], cv2.COLOR_BGR2RGB))
plt.title(‘first Segmented Frame’)
plt.axis(‘off’)
plt.show()
We get the following results:
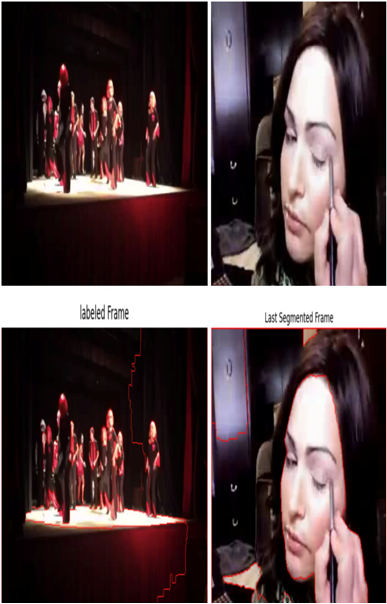
Figure 9.7 – The original, labeled, and segmented frames
The Watershed algorithm is a powerful tool in image segmentation, capable of handling complex and challenging segmentation tasks. In this section, we have introduced the Watershed algorithm, explaining its principles, steps, and applications. By understanding the underlying concepts and techniques, you will be equipped with the knowledge to apply the Watershed algorithm to segment and label video data effectively. Whether it is for medical imaging, quality control, or video analysis, the Watershed algorithm offers a versatile and reliable solution for extracting meaningful regions and objects from images and videos. Now, let’s see some real-world examples in the industry using video data labeling.
The Watershed algorithm is a region-based segmentation technique that operates on grayscale images. Its computational complexity depends on several factors, including the size of the input image, the number of pixels, and the characteristics of the image itself.
Leave a Reply